TriOsc
A source unit that can generate a range of triangle-shaped signals such as the
triangle wave and the
sawtooth wave. The signal can be
adjusted by changing the period
and/or frequency
of the oscillation.
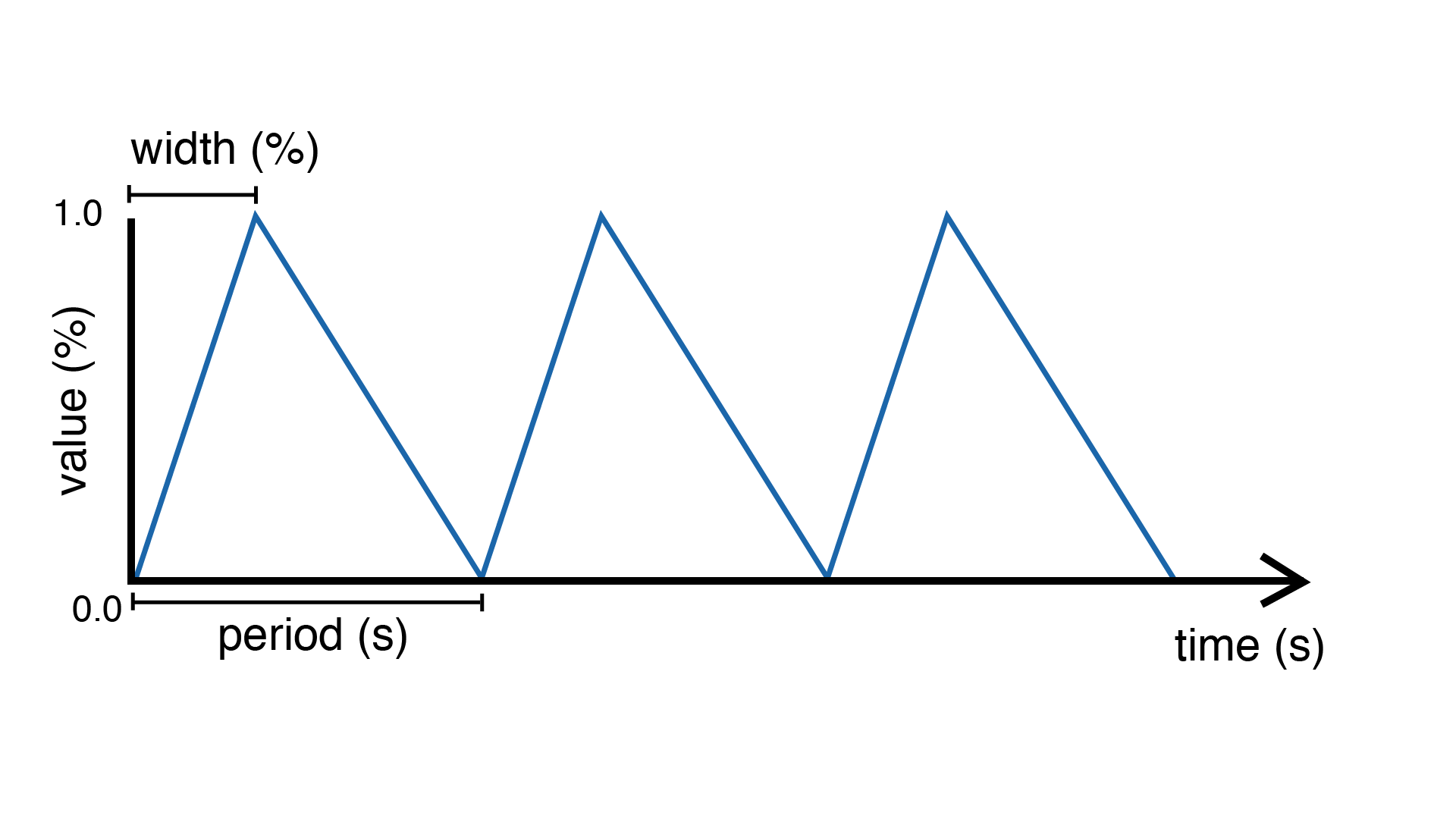
The width
parameter represents the “turning point” during the period at which
the signals reaches its maximum and starts going down again. Changing the width
allows to generate different kinds of triangular-shaped waves. For example, by
setting width to 1.0 (100%) one obtains a sawtooth wave; by setting it to 0.0 (0%)
an inverted sawtooth is created; anything in between generates different flavors
of triangle waves.
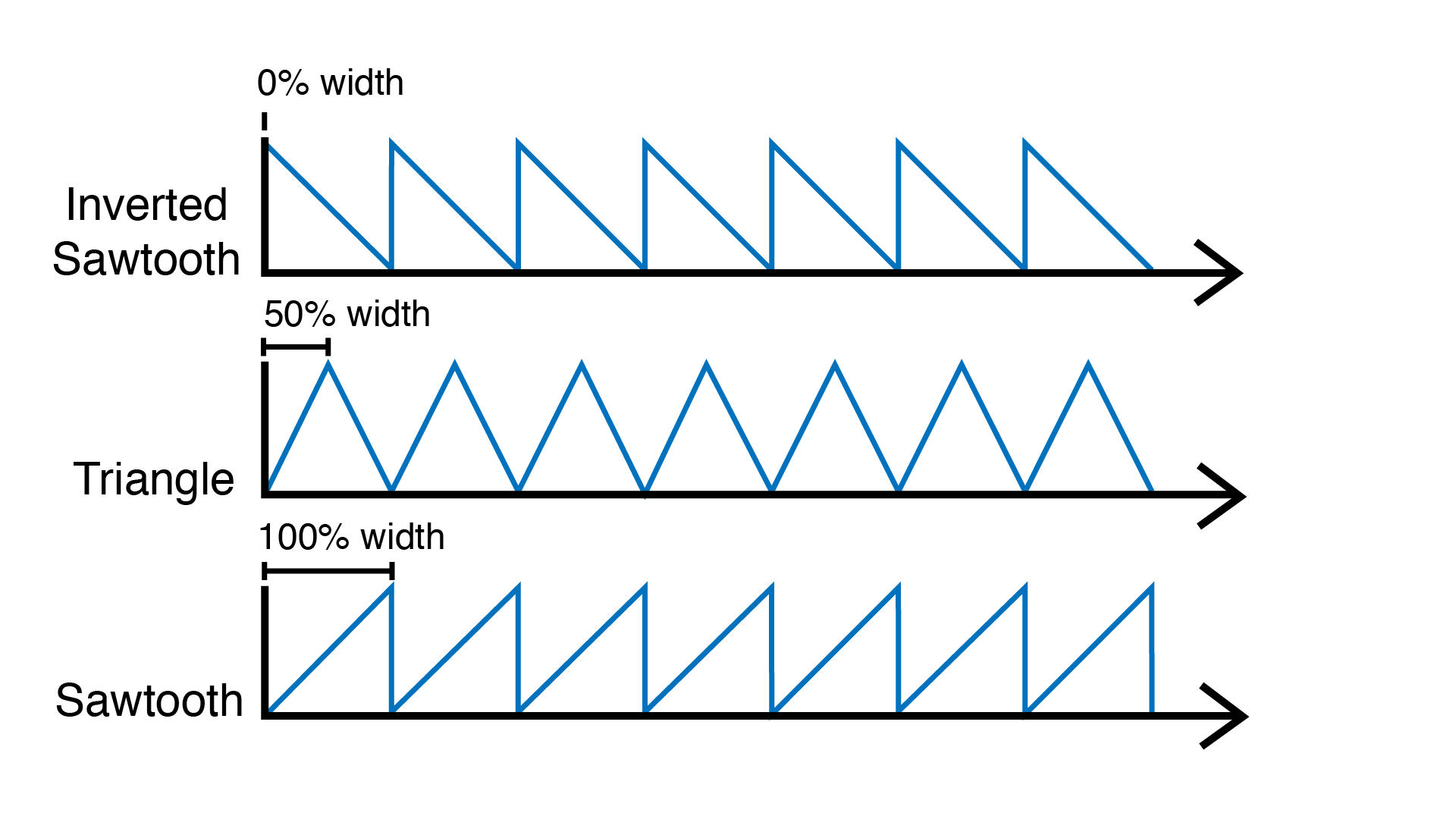
Example
Controls a set of traffic lights that go: red, yellow, green, red, yellow, green, and so on. It uses a sawtooth to iterate through these three states.
#include <Plaquette.h>
DigitalOut green(10);
DigitalOut yellow(11);
DigitalOut red(12);
TriOsc osc(10.0);
void begin() {
osc.width(1.0); // sawtooth wave
}
void step() {
// Shut down all lights.
0 >> led >> yellow >> green;
// Switch appropriate LED.
if (osc < 0.4)
green.on();
else if (osc < 0.6)
yellow.on();
else
red.on();
}
-
class TriOsc : public Osc
Triangle/sawtooth oscillator.
Public Functions
-
TriOsc(float period = 1.0f, float width = 0.5f)
Constructor.
- Parameters:
period – the period of oscillation (in seconds)
width – a value in [0, 1] that determines the point at which the wave reaches its maximum point (expressed as a fraction of the period)
-
virtual void width(float width)
Sets the width of the wave.
- Parameters:
width – a value in [0, 1] that determines the point at which the wave reaches its maximum point (expressed as a fraction of the period)
- Returns:
the unit itself
-
inline virtual float width() const
Returns the width of the wave.
-
virtual void period(float period)
Sets the period (in seconds).
- Parameters:
period – the period of oscillation (in seconds)
-
virtual void frequency(float frequency)
Sets the frequency (in Hz).
- Parameters:
frequency – the frequency of oscillation (in Hz)
-
inline virtual float frequency() const
Returns the frequency (in Hz).
-
virtual void amplitude(float amplitude)
Sets the amplitude of the wave.
- Parameters:
amplitude – a value in [0, 1] that determines the amplitude of the wave (centered at 0.5).
-
inline virtual float amplitude() const
Returns the amplitude of the wave.
-
virtual void phase(float phase)
Sets the phase (ie.
the offset, in % of period).
- Parameters:
phase – the phase (in % of period)
-
inline virtual float phase() const
Returns the phase (in % of period).
-
inline virtual float get()
Returns value in [0, 1].
-
TriOsc(float period = 1.0f, float width = 0.5f)