Ramp
A source unit that generates a smooth transition between two values. The unit can be triggered to start transitioning to a target value for a certain duration.
There are two ways to start the ramp.
By calling start(from, to, duration)
the ramp will transition from value from
to
value to
in duration
seconds.
Alternatively, calling start(to, duration)
will start a transition from the ramp’s
current value to to
in duration
seconds.
The following diagram shows what happens to the ramp signal if start(5.0, 1.0, 2.0)
is
called, followed later by start(3.0, 1.0)
:
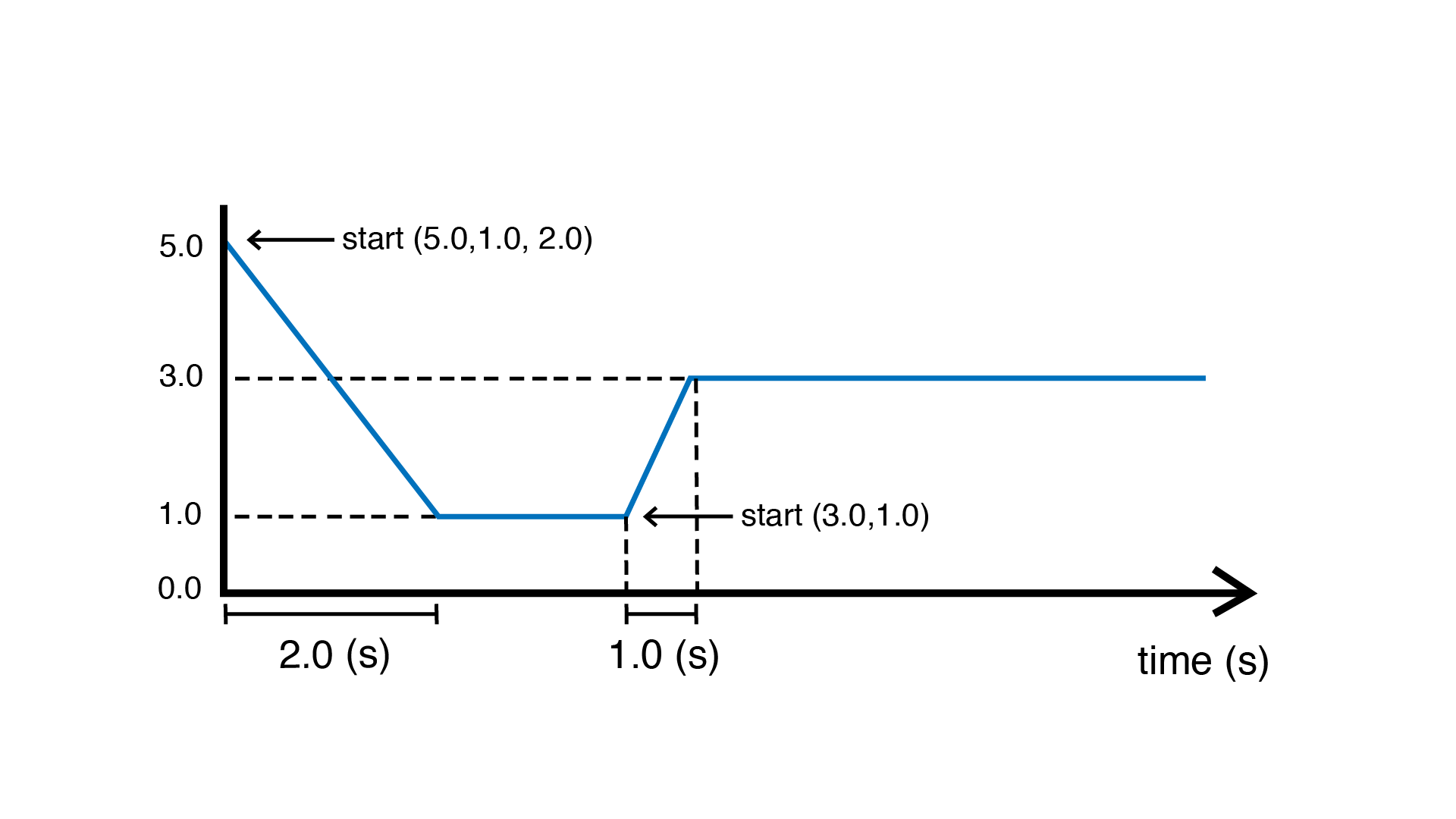
Note
Ramps also support the use of easing functions in order to
create different kinds of expressive effects with signals. An easing function can
optionally be specified at the end of a start()
command or by calling the
easing()
function.
Please refer to this page for a full list of available easing functions.
Example
Sequentially ramps through different values.
#include <Plaquette.h>
Ramp myRamp(0.0); // the ramp is initialized at zero (0)
StreamOut serialOut(Serial);
void begin() {
// Apply an easing function (optional).
myRamp.easing(easeOutSine);
}
void step() {
if (myRamp.isFinished())
{
// Restarts the ramp going from current value to a random value in [-10, +10] in 2 seconds
myRamp.start(randomFloat(-10, 10), 2.0);
}
myRamp >> serialOut;
}
Reference
-
class Ramp : public Node, public AbstractTimer
Provides a ramping / tweening mechanism that allows smooth transitions between two values.
Public Functions
-
Ramp(float from = 0.0f)
Basic constructor.
Use one of the start(…) functions to launch ramps with specific parameters.
- Parameters:
from – the value the ramp starts with
-
Ramp(float from, float to, float duration, easing_function easing = easeNone)
Basic constructor.
Use one of the start(…) functions to launch ramps with specific parameters.
- Parameters:
from – the initial value
to – the final value
duration – the duration of the ramp (in seconds)
easing – the easing function to apply (default: no easing)
-
inline virtual float get()
Returns value of ramp.
-
void easing(easing_function easing)
Sets easing function to apply to ramp.
- Parameters:
easing – the easing function
-
inline void noEasing()
Remove easing function (linear/no easing).
-
virtual void to(float to)
Assign final value of the ramp starting from current value.
- Parameters:
to – the final value
-
virtual void fromTo(float from, float to)
Assign initial and final values of the ramp.
- Parameters:
from – the initial value
to – the final value
-
virtual void start()
Starts/restarts the ramp. Will repeat the last ramp.
-
virtual void start(float to, float duration, easing_function easing = 0)
Starts a new ramp, starting from current value.
- Parameters:
to – the final value
duration – the duration of the ramp (in seconds)
easing – the easing function (optional)
-
virtual void start(float from, float to, float duration, easing_function easing = 0)
Starts a new ramp.
- Parameters:
from – the initial value
to – the final value
duration – the duration of the ramp (in seconds)
easing – the easing function (optional).
-
virtual void start(float duration)
Starts/restarts the chronometer with specific duration.
-
virtual float progress() const
The progress of the timer process (in %).
-
inline virtual bool isFinished() const
Returns true iff the chronometer has completed its process.
-
virtual void stop()
Interrupts the chronometer.
-
virtual void resume()
Resumes process.
-
inline virtual float elapsed() const
The time currently elapsed by the chronometer (in seconds).
-
inline bool isStarted() const
Returns true iff the chronometer is currently running.
-
Ramp(float from = 0.0f)