SquareOsc
A source unit that generates a square wave
signal. The signal can be tuned by changing the period
and/or frequency
of the oscillation, as well as the duty cycle
.
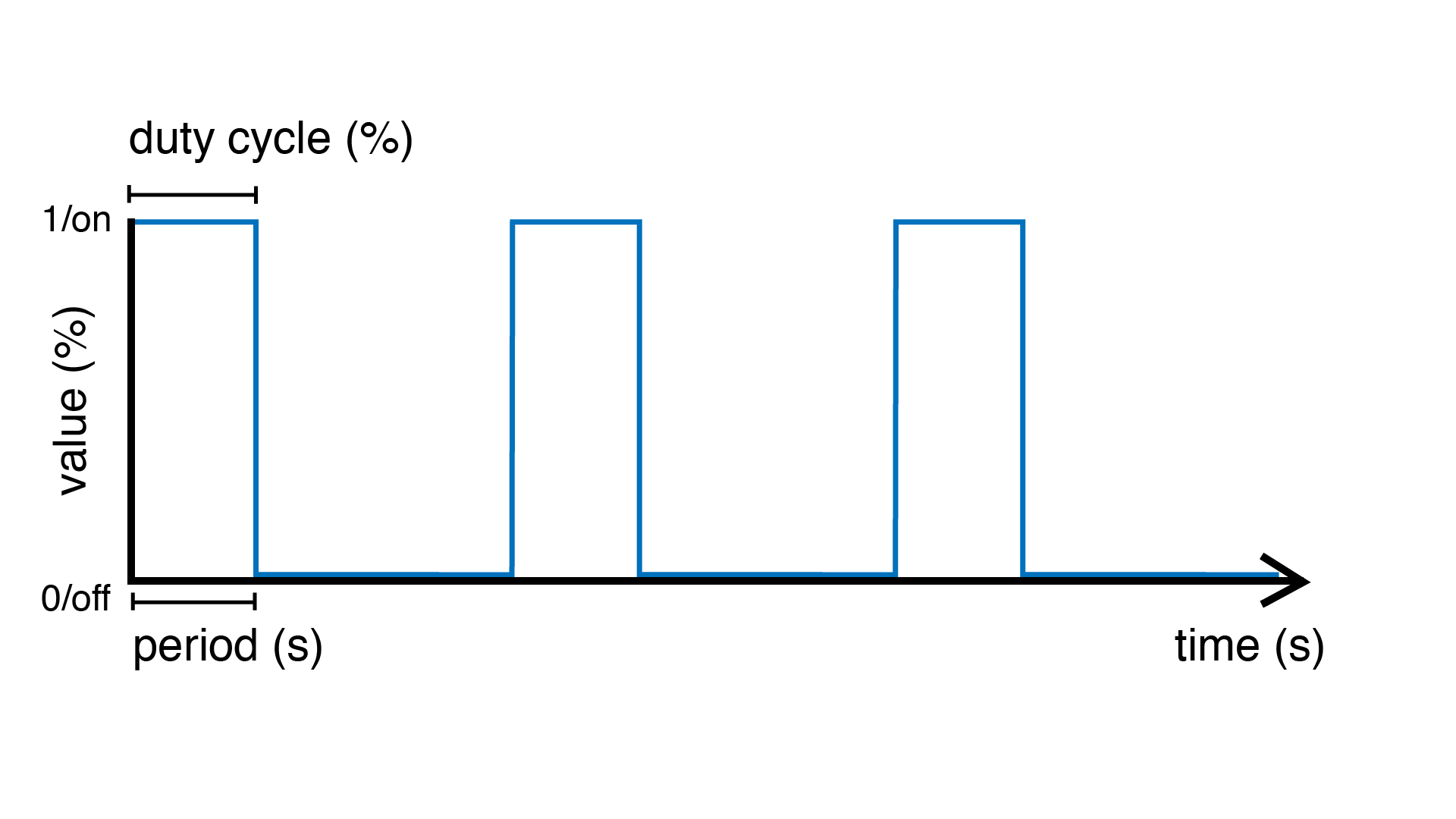
The duty cycle represents the proportion of time (expressed as a percentage) in each cycle (period) during which the wave is “on”.
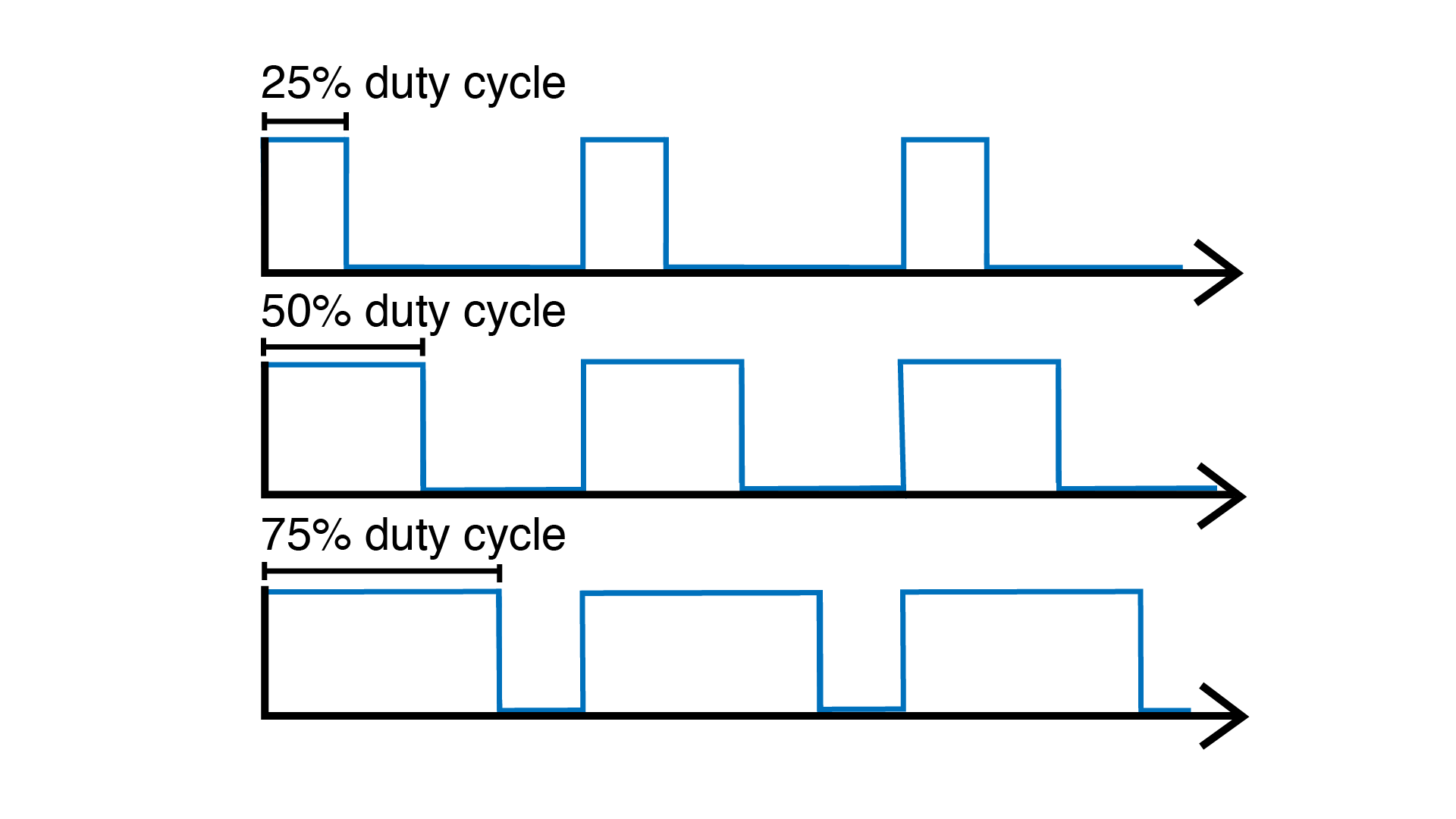
Example
Makes the built-in LED blink with a period of 4 seconds. Because the duty cycle is set to 25%, the LED will stay on for 1 second and then off for 3 seconds.
#include <Plaquette.h>
DigitalOut led(13);
SquareOsc blinkOsc(4.0);
void begin() {
blinkOsc.dutyCycle(0.25); // Sets the duty cycle to 25%
}
void step() {
blinkOsc >> led;
}
-
class SquareOsc : public Osc
Square oscillator. Duty cycle is expressed as % of period.
Public Functions
-
SquareOsc(float period = 1.0f, float dutyCycle = 0.5f)
Constructor.
- Parameters:
period – the period of oscillation (in seconds)
dutyCycle – the duty-cycle as a value in [0, 1]
-
virtual void dutyCycle(float dutyCycle)
Sets the duty-cycle (ie.
the proportion of time during which the signal is on).
- Parameters:
dutyCycle – the duty-cycle as a value in [0, 1]
-
inline virtual float dutyCycle() const
Returns the duty-cycle (as a value in [0, 1]).
-
virtual void period(float period)
Sets the period (in seconds).
- Parameters:
period – the period of oscillation (in seconds)
-
virtual void frequency(float frequency)
Sets the frequency (in Hz).
- Parameters:
frequency – the frequency of oscillation (in Hz)
-
inline virtual float frequency() const
Returns the frequency (in Hz).
-
virtual void amplitude(float amplitude)
Sets the amplitude of the wave.
- Parameters:
amplitude – a value in [0, 1] that determines the amplitude of the wave (centered at 0.5).
-
inline virtual float amplitude() const
Returns the amplitude of the wave.
-
virtual void phase(float phase)
Sets the phase (ie.
the offset, in % of period).
- Parameters:
phase – the phase (in % of period)
-
inline virtual float phase() const
Returns the phase (in % of period).
-
inline virtual float get()
Returns value in [0, 1].
-
SquareOsc(float period = 1.0f, float dutyCycle = 0.5f)