Smoother
Smooths the incoming signal by removing fast variations and noise (high frequencies).
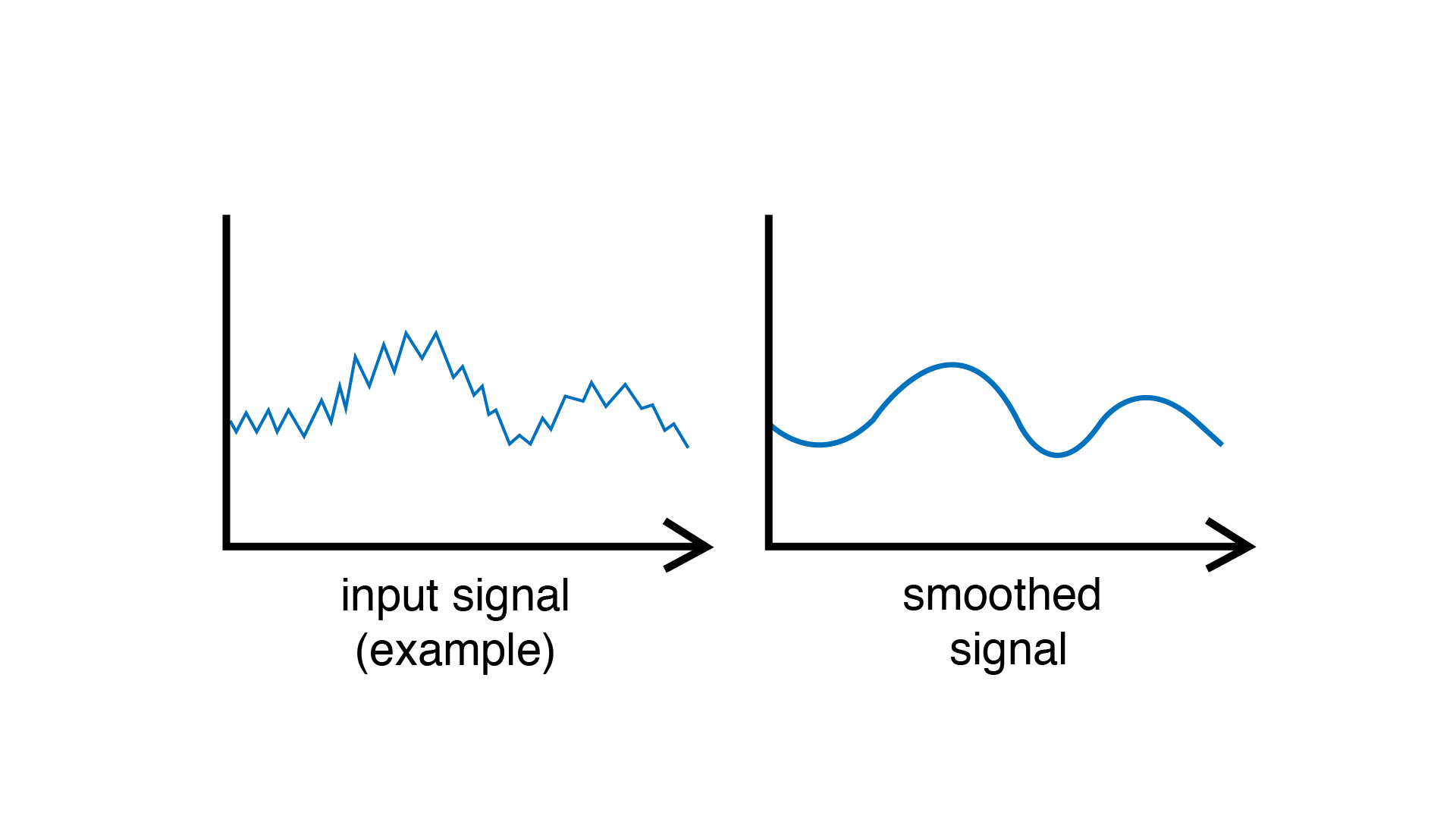
Example
Smooth a sensor over time.
#include <Plaquette.h>
AnalogIn sensor(A0);
// Smooths over time window of 10 seconds.
Smoother smoother(10.0);
StreamOut serialOut(Serial);
void begin() {}
void step() {
// Smooth value and send it to serial output.
sensor >> smoother >> serialOut;
}
Note
The filter uses an exponential moving average which corresponds to a form of low-pass filter.
Reference
-
class Smoother : public Unit, public MovingAverage
Simple moving average transform filter.
Public Functions
-
Smoother(Engine &engine = Engine::primary())
Constructor with default smoothing.
- Parameters:
engine – the engine running this unit
-
Smoother(float smoothingWindow, Engine &engine = Engine::primary())
Constructor with smoothing window.
- Parameters:
smoothingWindow – the time window over which the smoothing applies (in seconds)
engine – the engine running this unit
-
virtual float put(float value)
Pushes value into the unit.
- Parameters:
value – the value sent to the unit
- Returns:
the new value of the unit
-
inline virtual float get()
Returns smoothed value.
-
void timeWindow(float seconds)
Changes the smoothing window (expressed in seconds).
-
inline float timeWindow() const
Returns the smoothing window (expressed in seconds).
-
void cutoff(float hz)
Changes the smoothing window cutoff frequency (expressed in Hz).
-
float cutoff() const
Returns the smoothing window cutoff frequency (expressed in Hz).
-
Smoother(Engine &engine = Engine::primary())