PeakDetector
This unit detects peaks (minima or maxima) in an incoming signal. Peaks are detected based on crossing a trigger threshold above (or below) which a peak is detected.
Two different ways are supported to do this:
In crossing modes (
PEAK_RISING
andPEAK_FALLING
) the peak is detected as soon as the signal crosses thetriggerThreshold
.In apex modes (
PEAK_MAX
andPEAK_MIN
) the peak is detected after the signal crosses thetriggerThreshold
, reaches its apex, and then falls back by a certain proportion (%) between the threshold and the apex (controlled by thefallbackTolerance
parameter).
In all cases, after a peak is detected, the detector will wait until the signal
crosses back the reloadThreshold
(which can be adjusted to control detection
sensitivity) before it can be triggered again.
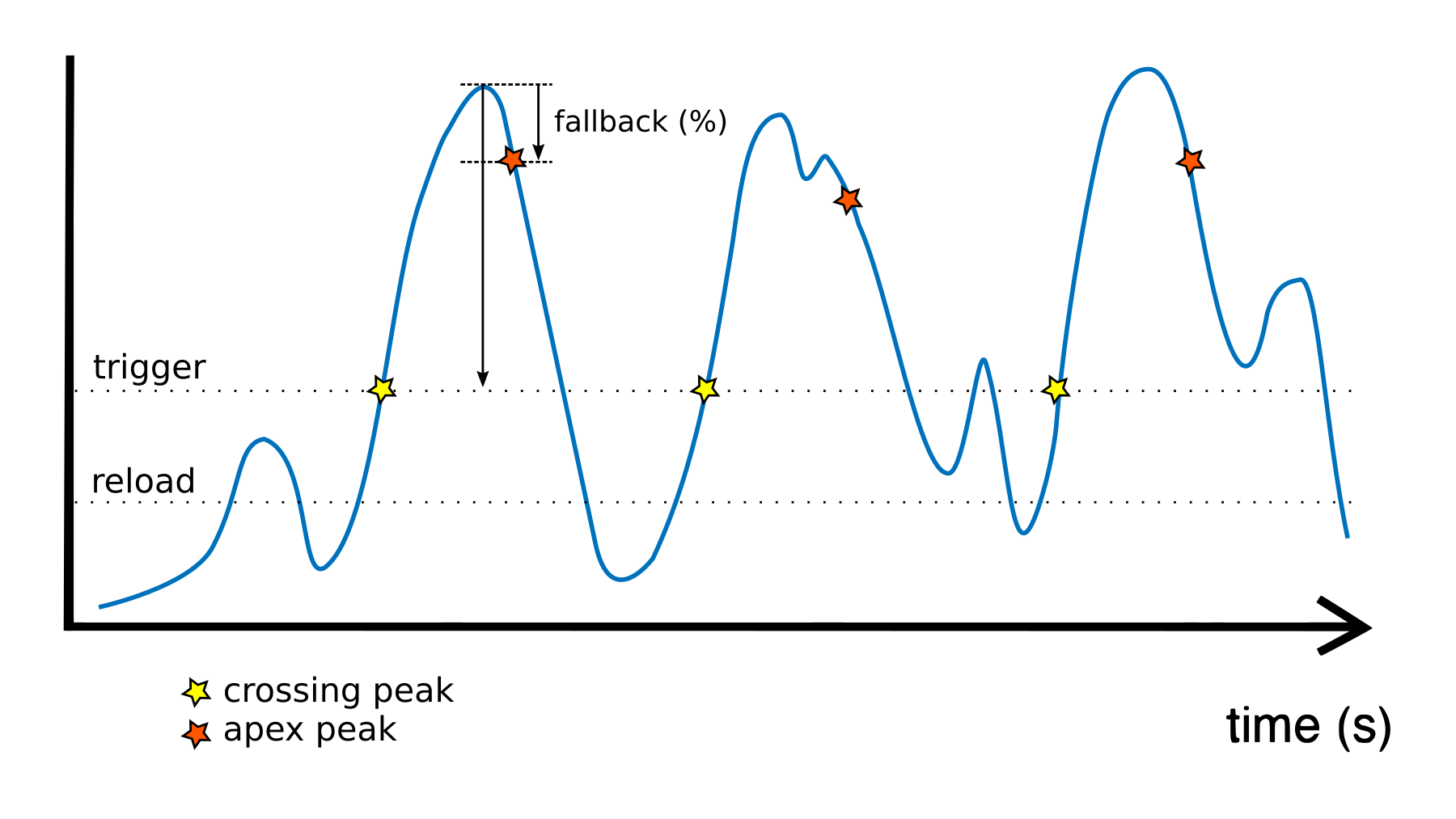
In summary, the four different modes available are:
PEAK_RISING
: peak detected as soon asvalue >= triggerThreshold
, then wait untilvalue < reloadThreshold
PEAK_FALLING
: peak detected as soon asvalue <= triggerThreshold
, then wait untilvalue > reloadThreshold
PEAK_MAX
: peak detected aftervalue >= triggerThreshold
and then falls back after peaking; then waits untilvalue < reloadThreshold
PEAK_MIN
: peak detected aftervalue <= triggerThreshold
and then falls back after peaking; then waits untilvalue > reloadThreshold
Note
Before sending a signal to a PeakDetector unit, it is recommended to normalize
signals, preferably using the Normalizer unit. Furthermore, to avoid a
noisy signal to generate false peaks, it is recommended to smooth the signal
by calling the source unit’s smooth()
method or by using a Smoother
unit.
Example
Uses a Normalizer and a PeakDetector to analyze input sensor values and detect peaks. Toggle and LED each time a peak is detected.
#include <Plaquette.h>
// Analog sensor (eg. photocell or microphone).
AnalogIn sensor(A0);
// Normalization unit to normalize values.
Normalizer normalizer;
// Peak detector. Threshold is set at 1.5 standard deviations above normal.
PeakDetector detector(normalizer.highOutlierThreshold(1.5)); // default mode = PEAK_MAX
// NOTE: You can change mode using optional 2nd parameter, example:
// PeakDetector detector(1.5, PEAK_FALLING));
// Digital LED output.
DigitalOut led;
void begin() {
// Adjust reload threshold to smaller value than reloadThreshold.
detector.reloadThreshold(normalizer.highOutlierThreshold(1.0));
// Adjust fallback tolerance as % between apex and trigger threshold.
detector.fallbackTolerance(0.2); // 0.2 = 20% (default: 10%)
// Smooth signal to avoid false peaks due to noise.
sensor.smooth();
// Set a time window of 1 minute (60 seconds) on normalizer.
// This will allow the normalier to slowly readjust itself
// if the lighting conditions change.
normalizer.timeWindow(60.0f);
};
void step() {
// Signal is normalized and sent to peak detector.
sensor >> normalizer >> detector;
// Toggle LED when peak detector triggers.
if (detector)
led.toggle();
}
Reference
-
class PeakDetector : public DigitalNode
Emits a signals when a signal peaks.
Public Functions
-
PeakDetector(float triggerThreshold, uint8_t mode = PEAK_MAX)
Constructor.
Possible modes are:
PEAK_RISING : peak detected when value becomes >= triggerThreshold, then wait until it becomes < reloadThreshold (*)
PEAK_FALLING : peak detected when value becomes <= triggerThreshold, then wait until it becomes > reloadThreshold (*)
PEAK_MAX : peak detected after value becomes >= triggerThreshold and then falls back after peaking; then waits until it becomes < reloadThreshold (*)
PEAK_MIN : peak detected after value becomes <= triggerThreshold and then rises back after peaking; then waits until it becomes > reloadThreshold (*)
- Parameters:
triggerThreshold – value that triggers peak detection
mode – peak detection mode
-
void triggerThreshold(float triggerThreshold)
Sets triggerThreshold.
-
inline float triggerThreshold() const
Returns triggerThreshold.
-
void reloadThreshold(float reloadThreshold)
Sets minimal threshold that “resets” peak detection in crossing (rising/falling) and peak (min/max) modes.
-
inline float reloadThreshold() const
Returns minimal value “drop” for reset.
-
void fallbackTolerance(float fallbackTolerance)
Sets minimal relative “drop” after peak to trigger detection in peak (min/max) modes, expressed as proportion (%) of peak minus triggerThreshold.
-
inline float fallbackTolerance() const
Returns minimal relative “drop” after peak to trigger detection in peak modes.
-
bool modeInverted() const
Returns true if mode is PEAK_FALLING or PEAK_MIN.
-
bool modeCrossing() const
Returns true if mode is PEAK_RISING or PEAK_FALLING.
-
void mode(uint8_t mode)
Sets mode.
-
inline uint8_t mode() const
Returns mode.
-
virtual float put(float value)
Pushes value into the unit.
- Parameters:
value – the value sent to the unit
- Returns:
the new value of the unit
-
inline virtual bool isOn()
Returns true iff the triggerThreshold is crossed.
-
inline virtual float get()
Returns value as float (either 0.0 or 1.0).
-
PeakDetector(float triggerThreshold, uint8_t mode = PEAK_MAX)